1.28-round-lcd-breakout
From SB-Components Wiki
1.28" Round LCD Breakout
The 1.28" Round LCD Breakout is a 1.28-inch display breakout with 240 x 240 resolution, 65K RGB colours, and a clear and colourful displaying effect meant to help gadgets increase their interaction via SPI connection by providing a GPIO header for easy interface. The GC9A01 display driver and SPI interface are integrated into the 1.28" Round LCD Breakout, decreasing the number of IO pins required.
Features
- 4-wire SPI Communication
- GPIO header for easy interfacing
- Cross-Platform compatibility
- 1.28” Round LCD
Specifications
- Operating Voltage - 3.3 V
- Pixel Size - 0.135 × 0.135mm
- Board Dimension - 36mm x 44mm
- Pixels - 240×240 resolution
- Display Colour - 65K RGB
- Driver - GC9A01
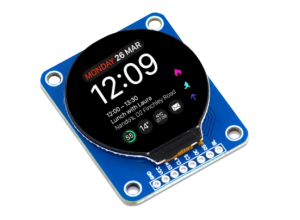
Pinout
Round LCD Breakout | Description |
---|---|
GND | Ground Supply |
VCC | Power Supply 3.3V - 5V DC |
DIN | Serial Data Input / Output |
CLK | Serial Clock |
CS | Chip select input pin ("Low" enable) |
DC | Display data / Command selection pin DC='1': Display data. DC='0': Command data |
RST | Reset Pin. Initialize the chip with a low input |
BL | Blacklight Control. High: Blacklight on Low: Blacklight off. |
Installation
Arduino
If you are using Arduino Ide, you can use the above library.
PICO RP2040 (MicroPython)
- Below components are required to run Pico RP2040 compatible boards :
- Raspberry Pi Pico x 1
- Pico 1.28" Round LCD Breakout x 1
- USB Cable x 1
- Copy or clone code from Github.
https://github.com/sbcshop/1.28-Round-LCD-Breakout
- Connect LCD breakout with Raspberry Pi Pico as shown in the below pinout.
1.28" Round LCD Breakout | PICO Pins |
---|---|
VCC | 3.3V / 5V |
GND | GND |
LCD DIN | GP11 |
LCD CLK | GP10 |
LCD DC | GP8 |
LCD CS | GP9 |
LCD RST | GP12 |
LCD BL | GP13 |
- Press and hold BOOTSEL pin of Raspberry Pi Pico and connect the USB cable, it will create a drive as RPI-RP2.
- Now drag and drop or copy and paste firmware.uf2 file from firmware folder to RPI-RP2 Drive. It will reboot your raspberry pi pico.
- Open Thonny IDE and Choose interpreter as MicroPython (Raspberry Pi Pico).
- Now you can run example codes from the Pico_Example_mpy folder in Thonny Ide. For example, Open the hello.py file in thonny and click on the green play button to run this example.
It will print "Hello" on Round LCD. You can use the joystick to change the LCD color.
Working Example
- This module was tested on Raspberry Pi Pico. You need to provide a SPI object and the pin to use for the DC pin of the screen.
import machine import gc9a01 spi = SPI(1, baudrate=40000000, sck=Pin(10), mosi=Pin(11)) tft = gc9a01.GC9A01(spi, 240, 240, reset=Pin(12, Pin.OUT), cs=Pin(9, Pin.OUT), dc=Pin(8, Pin.OUT), backlight=Pin(13, Pin.OUT), rotation=0) tft.init()
Functions and Arguments
gc9a01.GC9A01(spi, width, height, reset, dc, cs, backlight, rotation, buffer_size)
required arguments:
`spi` spi device `width` display width `height` display height
optional args:
`reset` reset pin `dc` dc pin `cs` cs pin `backlight` backlight pin `rotation` Orientation of display. `buffer_size` 0= buffer dynamically allocated and freed as needed. Rotation | Orientation -------- | -------------------- 0 | 0 degrees 1 | 90 degrees 2 | 180 degrees 3 | 270 degrees 4 | 0 degrees mirrored 5 | 90 degrees mirrored 6 | 180 degrees mirrored 7 | 270 degrees mirrored
If buffer_size is specified it must be large enough to contain the largest bitmap, font character, and/or JPG used (Rows * Columns *2 bytes). Specifying a buffer_size reserves memory for use by the driver otherwise, memory required is allocated and free dynamically as it is needed. Dynamic allocation can cause heap fragmentation so garbage collection (GC) should be enabled.
This driver supports only 16bit colors in RGB565 notation.
GC9A01.on()
Turn on the backlight pin if one was defined during init.
GC9A01.off()
Turn off the backlight pin if one was defined during init.
GC9A01.pixel(x, y, color)
Set the specified pixel to the given color.
GC9A01.line(x0, y0, x1, y1, color)
Draws a single line with the provided color from (x0, y0) to (x1, y1).
GC9A01.hline(x, y, length, color)
Draws a single horizontal line with the provided color and length in pixels. Along with vline, this is a fast version with reduced number of SPI calls.
GC9A01.vline(x, y, length, color)
Draws a single horizontal line with the provided color and length in pixels.
GC9A01.rect(x, y, width, height, color)
Draws a rectangle from (x, y) with corresponding dimensions
GC9A01.fill_rect(x, y, width, height, color)
Fill a rectangle starting from (x, y) coordinates
Source code credit: https://github.com/russhughes/gc9a01_mpy
Resources
Github
Datasheet